LibreTranslate - Free and Open Source Machine Translation API
LibreTranslate - Free and Open Source Machine Translation API
https://libretranslate.com/
Free and Open Source Machine Translation API. Self-hosted, offline capable and easy to setup. Run your own API server in just a few minutes.
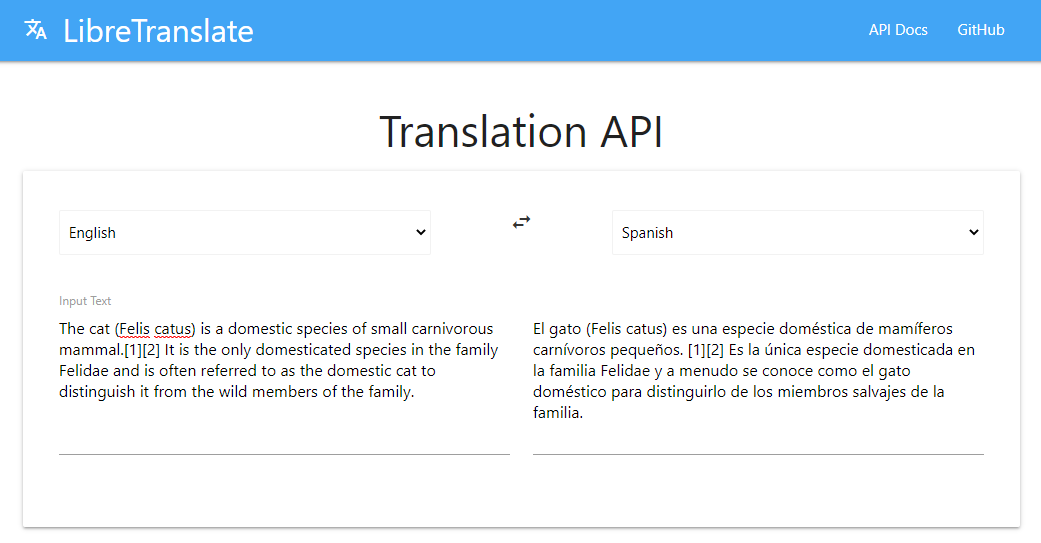
Karma FW | Internet FireWall
Ever wanted to block an app from using the internet? Why does my keyboard need access to the internet? Can i block ads in this game when it has no internet access?
There are many reasons one might want to block an app from using the internet. Recently i came across a FOSS app that acts as a proxy and only allows selected apps from passing through and connecting to the internet. Set it up once and let it run from boot automatically after setup.
Unlike Xiaomi devices, sadly there are few Android skins that supports this feature out of the box. This app brings it to all.
ragedev | devRant Clone
I messed up with the concept of this competition and created a client instead of a clone. It uses devRant api and displays the output with an HTML template.
here's my GitHub repo link - https://github.com/sidaims93/ragedev
Tech stack I used -
Laravel HTML CSS JS MySQL
It took me a total of 5 hours
Nothing special about this lol.
You can also watch a livestream of mine where I worked on it - https://youtube.com/live/nu0UREIMRSQ?feature=share
Watch it and if you like, hit the like button and Subscribe!
[\#introduction](https://kbin.melroy.org/tag/introduction)
Software Developer from Canada
Played video games and hacked things a bit as a teenager, got a computer science degree and worked in corporate / consulting
Specialised in Java, liked JavaScript much more, now bashing my brains against #rust and moving in
I have a bunch of hobbies and like exploring weird out of the way things. Interest in psychology, #economics, business, and systems as a general.
Hi everyone!
Hi everyone!
I'm nearly ready to release an Alpha version of my Prototype Editor. It allows you to create mock-ups of things like websites. You can find my first proper YT video here: https://www.youtube.com/watch?v=6o2Ho8qhMAI - please like, comment and sub if you can. It will help motivate me more and will help support the project.
How to create a lexer using pure C - part 1
How to write a lexer in C - part 1
A lexer translates text to tokens which you can use in your application. With these tokens your could build your own programming language or a json parser for example. Since i'm fan of OOP you'll see that I applied an OO aproach. The code quality is good according to ChatGPT. According to valgrind there are no memory leaks. We're gonna create in this part:
- token struct
- token tests
- Makefile
Requirements:
- gcc
- make
Writing token.h
Create a new file called "token.h".
Implement header protection:
#ifndef TOKEN_H_INCLUDED
#define TOKEN_H_INCLUDED
// Where the code goes
#endif
This will prevent that the file gets double included.
Import the headers we need:
#include <string.h>
#include <stdlib.h>
Define config:
#define TOKEN_LEXEME_SIZE 256
this means our token size is limited to 256 chars. It's not possible to use a huge string now. Dynamic memory allocation is too much to include in this tutorial.
Define the token struct:
typedef struct Token {
char lexeme[TOKEN_LEXEME_SIZE];
int line;
int col;
struct Token * next;
struct Token * prev;
} Token;
Implement new function. This will instantiate Token with default values.
Token * token_new(){
Token * token = (Token *)malloc(sizeof(Token));
memset(token->lexeme, 0,TOKEN_LEXEME_SIZE);
token->line = 0;
token->col = 0;
token->next = NULL;
token->prev = NULL;
return token;
}
Imlement init function. This will instantiate a Token with given parameters as values.
Token * token_init(Token * prev, char * lexeme, int line, int col){
Token * token = token_new();
token->line = line;
token->col = col;
if(prev != NULL){
token->prev = prev;
prev->next = token;
}
strcpy(token->lexeme, lexeme);
return token;
}
Implement free function. This is our destructor.
It will:
- find first token using given token
- will call itself with related token(s)
void token_free(Token * token){
// Find first token
while(token->prev != NULL)
token = token->prev;
Token * next = token->next;
if(next){
token->next->prev = NULL;
token_free(next);
}
free(token);
}
Testing
Now it's time to build some tests using assert.
Create a new file called "token_test.h".
Add this:
int main()
{
// Check default values
Token *token = token_new();
assert(token->next == NULL);
assert(token->prev == NULL);
assert(token->line == 0);
assert(token->col == 0);
assert(strlen(token->lexeme) == 0);
// Test init function
Token *token2 = token_init(token, "print", 1, 3);
assert(token->next == token2);
assert(token2->prev == token);
assert(token2->line == 1);
assert(token2->col == 3);
assert(!strcmp(token2->lexeme, "print"));
token_free(token2);
printf("Tests succesful\n");
return 0;
}
Now we have a working application. Let's make compilation easy using a Makefile.
Makefile
Create a file named "Makefile".
all: tests
tests:
gcc token_test.c -o token_test
./token_test
Run make
That's it!
So, we created Token which is required for the lexer in next part of this tutorial.
If something not working or you need help; send a message.