Are there better alternatives to null-terminated strings?
fatal error: dbus/dbus-arch-deps.h: No such file or directory
im new to system programming, idk if thats the issuse. but according gcc, it can not find dbus/dbus-arch-deps.h
and thats all i know. any idea about this issue?
program
[I] tomri@artix ~ [1]> cat main.c
#include <dbus-1.0/dbus/dbus.h>
int main(void) { return 0; }
# error
[I] tomri@artix ~> gcc main.c
In file included from main.c:1:
/usr/include/dbus-1.0/dbus/dbus.h:29:10: fatal error: dbus/dbus-arch-deps.h: No such file or directory
29 | #include <dbus/dbus-arch-deps.h>
| ^~~~~~~~~~~~~~~~~~~~~~~
compilation terminated.
[I] tomri@artix ~> ls -la /usr/include/dbus-1.0/dbus/
total 216
drwxr-xr-x 2 root root 4096 Jul 2 20:26 ./
drwxr-xr-x 3 root root 4096 Jun 15 21:36 ../
-rw-r--r-- 1 root root 2809 Jan 14 06:17 dbus-address.h
-rw-r--r-- 1 root root 3470 Jan 14 06:17 dbus-bus.h
-rw-r--r-- 1 root root 27018 Jan 14 06:17 dbus-connection.h
-rw-r--r-- 1 root root 2909 Jan 14 06:17 dbus-errors.h
-rw-r--r-- 1 root root 22076 Jun 8 2023 dbus-glib-bindings.h
-rw-r--r-- 1 root root 2575 Jun 8 2023 dbus-glib-lowlevel.h
-rw-r--r-- 1 root root 14766 Jun 8 2023 dbus-glib.h
-rw-r--r-- 1 root root 8969 Jun 8 2023 dbus-gtype-specialized.h
-rw-r--r-- 1 root root 1464 Jun 8 2023 dbus-gvalue-parse-variant.h
-rw-r--r-- 1 root root 7246 Jan 14 06:17 dbus-macros.h
-rw-r--r-- 1 root root 1961 Jan 14 06:17 dbus-memory.h
-rw-r--r-- 1 root root 15259 Jan 14 06:17 dbus-message.h
-rw-r--r-- 1 root root 1810 Jan 14 06:17 dbus-misc.h
-rw-r--r-- 1 root root 3809 Jan 14 06:17 dbus-pending-call.h
-rw-r--r-- 1 root root 23956 Jan 14 06:17 dbus-protocol.h
-rw-r--r-- 1 root root 5412 Jan 14 06:17 dbus-server.h
-rw-r--r-- 1 root root 5392 Jan 14 06:17 dbus-shared.h
-rw-r--r-- 1 root root 3047 Jan 14 06:17 dbus-signature.h
-rw-r--r-- 1 root root 2359 Jan 14 06:17 dbus-syntax.h
-rw-r--r-- 1 root root 8505 Jan 14 06:17 dbus-threads.h
-rw-r--r-- 1 root root 4143 Jan 14 06:17 dbus-types.h
-rw-r--r-- 1 root root 3961 Jan 14 06:17 dbus.h
[I] tomri@artix ~>
# my system
[I] tomri@artix ~> uname --all
Linux artix 6.9.7-artix1-1 #1 SMP PREEMPT_DYNAMIC Fri, 28 Jun 2024 18:11:28 +0000 x86_64 GNU/Linux
open source structured thread safe logging library for C
Is there a library for C, providing thread safe (high performance), and structured logging? An example for rust is the Tracing crate for rust (from Tokio). It should support several outputs as well.
GNU C Library 2.38
https://lists.gnu.org/archive/html/info-gnu/2023-07/msg00010.html
Enforce same size for arrays at compile-time?
Let's say I have two arrays that have related data:
const char *backend_short[] = { "oal", "pa", "sdl_m" };
const char *backend_long[] = { "openal", "portaudio", "sdl_mixer" };
Does C support a way to "assert" that these two arrays have the same size? And failing compilation if they are different? I want a safeguard in case I'm drunk one day and forget to keep these synchronized.
Thanks in advance.
EDIT: I found a solution. Here are some enlightening resources on the matter:
Readline from stdin
If one has POSIX extensions available, then it seems that defining _POSIX_C_SOURCE and just using getline or detdelim is the way to go. However, for source that is just C, here is a solution I've found from various sources, mainly here. I've also tried to make it more safe.
// Read line from stdin
#include <stdio.h>
#include <stdlib.h>
#define CHUNK_SIZE 16 // Allocate memory in chunks of this size
// Safe self-realloc
void *xrealloc(void *ptr, size_t size)
{
void *tmp = realloc(ptr, size);
if (!tmp) free(ptr);
return tmp;
}
// Dynamically allocate memory for char pointer from stdin
char *readline(void)
{
size_t len = 0, chunk = CHUNK_SIZE;
char *str = (char *)malloc(CHUNK_SIZE);
if (!str) return NULL;
int ch;
while((ch = getchar()) != EOF && ch != '\n' && ch != '\r') {
str[len++] = ch;
if (len == chunk) {
str = (char *)xrealloc(str, chunk+=CHUNK_SIZE);
if (!str) return NULL;
}
}
str[len++] = '\0'; // Ensure str is null-terminated
return (char *)xrealloc(str, len);
}
int main(void)
{
setbuf(stdout, NULL); // Ensure environment doesn't buffer stdout
printf("Enter name: ");
char *userName = readline();
if (!userName) return 1;
printf("Hello, %s!\n", userName);
free(userName);
return 0;
}
The idea is that we allocate in chunks of 16, or whichever size. xrealloc is handy when reallocating a block of memory to itself.
int *ptr = malloc(sizeof(int) * 4);
ptr = (int *)realloc(ptr, sizeof(int) * 8);
If realloc fails, ptr gets assigned NULL
, and we lose the address that we need to free, causing a memory leak. So, xrealloc allows for safe self re-allocation.
Linus Torvalds on C++
Linus Torvalds on C++
http://harmful.cat-v.org/software/c++/linus
The Spirit of C
The Spirit of C ― Andreas Zwinkau
http://beza1e1.tuxen.de/articles/spirit_of_c.html
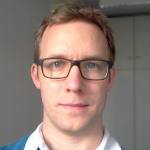
dwl is a dwm-like Wayland compositor based on wlroots
GitHub - djpohly/dwl: dwm for Wayland
https://github.com/djpohly/dwl
dwm for Wayland. Contribute to djpohly/dwl development by creating an account on GitHub.
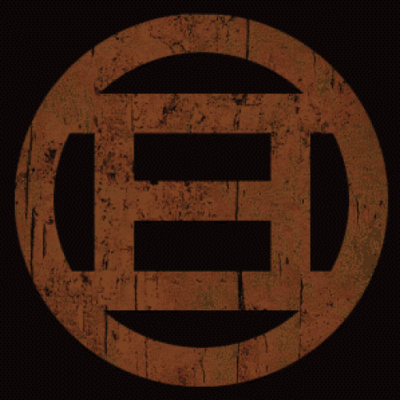
In browser JS to C compiler
In browser JS to C compiler
https://timr.co/in-browser-js-to-c-compiler